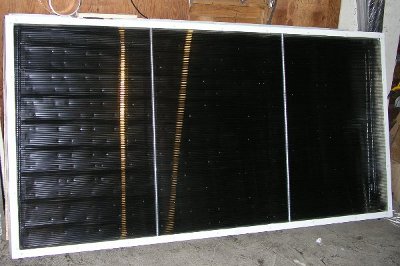
1. System Components
Below components are used for this project.
- Camera : will be connected to Receiver part using 2.5pi Audio Cable
- Receiver : Arduino Uno(MCU) + WizFi250 (Wi-Fi Module, using Soft AP mode),
- Remote Controller : Smart Phone (Simple Android App)
Connecting Canon DSLR camera with Receiver
We are going to use 2.5Ø audio jack to control the camera. As you see below image, the shutter and the focus will be controlled by this remote controller system.
If you take off the cover of the audio jack, you can see the red, white & gold wires. In this post, we are going to use the red line for shutter and white line for focus control. (You need to check which line you will use by using the Tester) The red and white lines will be connected to D7 & D6 of Arudino board respectively.
After then, connect the audio jack to the camera.
Programming for Receiver
We are going to use Soft AP function of WizFi250. In the Soft AP mode, WizFi250 operates as Access Point and supports direct connection with 2~3 devices.
Below is the code for Arduino. If ‘S’ is received from smart phone, the shutter operates to take a picture. If ‘F’ is received, it controls the focus.
#include <Arduino.h> #include <SPI.h> #include <IPAddress.h> #include "WizFi250.h" #include "WizFi250_tcp_server.h" #define SSID "600D_AP" #define KEY "123456789" #define AUTH "WPA2" #define FOCUS 6 #define SHUTTER 7 #define REMOTE_PORT 5000 #define LOCAL_PORT 5000 WizFi250 wizfi250; IPAddress ip(192,168,12,1); IPAddress subnet(255,255,255,0); IPAddress gateway(192,168,12,1); void pinSetup(); void PushFocus(); void ReleaseFocus(); void PushShutter(); void ReleaseShutter(); boolean Wifi_setup = false; WizFi250_TCP_Server myServer(LOCAL_PORT); String str; void setup() { Serial.begin(9600); Serial.println("\r\nSerial Init"); wizfi250.init(); wizfi250.setDebugPrint(4); wizfi250.hw_reset(); pinSetup(); wizfi250.sync(); wizfi250.setDhcp(ip,subnet,gateway); if( wizfi250.begin(SSID,KEY,AUTH,1) == RET_OK ) { Wifi_setup = true; } } void loop() { char cmd; if( Wifi_setup ) { wizfi250.RcvPacket(); if( myServer.isListen() != true ) { myServer.listen(); } if( myServer.available() ) { cmd = myServer.recv(); if( cmd == (uint8_t)'S') { ReleaseFocus(); PushShutter(); delay(500); ReleaseShutter(); } else if( cmd == (uint8_t) 'F') { ReleaseShutter(); PushFocus(); } cmd = NULL; } } } void pinSetup() { pinMode(FOCUS, OUTPUT); pinMode(SHUTTER, OUTPUT); digitalWrite(FOCUS, HIGH); digitalWrite(SHUTTER, HIGH); } void PushFocus() { digitalWrite(FOCUS, LOW); } void ReleaseFocus() { digitalWrite(FOCUS, HIGH); } void PushShutter() { digitalWrite(SHUTTER, LOW); } void ReleaseShutter() { digitalWrite(SHUTTER, HIGH); }
Smart Phone App Code
package com.example.canonremotecontrol; import android.support.v7.app.ActionBarActivity; import android.os.*; import android.util.Log; import android.view.*; import android.widget.*; import java.io.ObjectOutputStream; import java.io.OutputStream; import java.net.*; public class MainActivity extends ActionBarActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button btnFocus = (Button)findViewById(R.id.focus_btn); btnFocus.setOnClickListener(new Button.OnClickListener(){ public void onClick(View v){ ConnectThread thread = new ConnectThread("192.168.12.1"); thread.SetAction("F"); thread.start(); } }); Button btnTake = (Button)findViewById(R.id.shutter_btn); btnTake.setOnClickListener(new Button.OnClickListener(){ public void onClick(View v){ ConnectThread thread = new ConnectThread("192.168.12.1"); thread.SetAction("S"); thread.start(); } }); } class ConnectThread extends Thread{ String hostname; String m_action; public ConnectThread(String addr){ hostname = addr; } public void SetAction(String action){ m_action = action; } public void run(){ try{ int port = 5000; Socket sock = new Socket(hostname, port); OutputStream out = sock.getOutputStream(); out.write(m_action.getBytes()); out.flush(); sock.close(); } catch(Exception ex){ ex.printStackTrace(); } } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
Below is demonstration video
COMMENTS