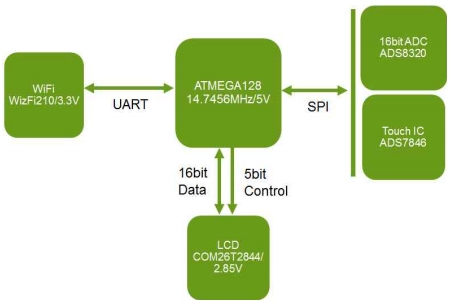
This example shows how to output time got from NTP time server to the serial monitor using the protocol of NTP (Network Time Protocol) application layer. NTP is a typical example using a UDP (User Datagram Protocol) transport protocol.
For more information: http: //en.wikipedia.org/wiki/Network_Time_Protocol
1. What is needed and the circuit configuration
Supplies
=====
Arduino Uno board(or Mega board)
Ethernet shield board
Internet router
Internet line
Circuit configuration
=======
Unnecessary Circuit configuration, but you should wire the Ethernet shield to Arduino Uno board and connect to the Internet.
2. sketch description
I modified the UdpNtpClient sketch provided by arduino Eno IDE, it was to be output together also South Korea time. If you want to use what is provided basically in arduio IDE, Choose “File -> example -> Ethernet-> UdpNtpClient”.
sketch
=====
/ *
Udp NTP Client (UDP NTP clients)
As an example, the packet information of time from the NTP (Network Time Protocol) the time the server time
Sketch
=====
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 | /* Udp NTP Client It shows how to send and receive UDP (User Datagram Protocol) packet data. It received the packet information of time from the NTP (Network Time Protocol) the time the server and outputs it to the serial monitor. More detailed information for the message to be each other exchanged and NTP time server Refer to the following: http: //en.wikipedia.org/wiki/Network_Time_Protocol Been creat ed by Michael Margolis on 09 May 04, 2010, Was fixed by Tom Igoe on 04 May 09, 2012 This code is the shared work. */ #include <SPI.h> #include <Ethernet.h> #include <EthernetUdp.h> // Please put the MAC address for the Ethernet controller // New Ethernet shield has MAC address is printed on a sticker byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED }; unsigned int localPort = 8888; // local port to receive a UDP packet char timeServer[] = “time.nist.gov”; // time.nist.gov NTP server const int NTP_PACKET_SIZE = 48; // size of NTP message(packet data) byte packetBuffer[ NTP_PACKET_SIZE]; // buffer for sending and receiving packet // UDP instance(object) for exchanging packet using protocol EthernetUDP Udp; void setup() { // Open a serial communication and wait for serial communication is connected: Serial.begin(9600); while (!Serial) { ; // Wait until the serial port is connected. Only Leonardo board is needed. } // start the UDP and Ehternet if (Ethernet.begin(mac) == 0) { Serial.println(“using DHCP, failed to configure the Ethernet”); // infinite loop : for (;;) ; } Udp.begin(localPort); } void loop() { sendNTPpacket(timeServer); // sends a NTP packet to the time server // Make sure that the receive response delay(1000); if ( Udp.parsePacket() ) { // Get data from received response packet Udp.read(packetBuffer, NTP_PACKET_SIZE); // Read the packet to buffer Unsigned long highWord = word (packetBuffer [40], packetBuffer [41]); Unsigned long lowWord = word (packetBuffer [42], packetBuffer [43]); // Combine 4 bytes (2 words) into a long type integer, // NTP time (seconds since January 1, 1900) // exchange): // The timestamp starts at the 40th byte of the received packet. consist of 4byte(2 word or long) // separate 2 word data. unsigned long highWord = word(packetBuffer[40], packetBuffer[41]); unsigned long lowWord = word(packetBuffer[42], packetBuffer[43]); // combine 4 byte(2word) and make it in long type integer // NTP time( calculate seconds since January 1, 1900 ) // unsigned long secsSince1900 = highWord << 16 | lowWord; Serial.print(“sec : “ ); Serial.println(secsSince1900); // Convert NTP time to daily time: Serial.print(“Unix time : “); // Unix time starts on January 1, 1970 and // I’ts 2208988800 calculated in seconds: const unsigned long seventyYears = 2208988800UL; // Subtract the corresponding seconds with 70 years: unsigned long epoch = secsSince1900 – seventyYears; // output Unix time: Serial.println(epoch); // output Universal Time Coordinated: Serial.print(“UTC : “); // ( UTC is the Greenwich // Meridian Time:GMT) int hour, minute, second; hour = (epoch % 86400L) / 3600;// 1 day = 86400 sec, 1h = 3600 sec minute = (epoch % 3600) / 60; // 1 h = 3600 sec, 1 minute = 60 sec second = epoch % 60; // 1 m = 60 sec printTime(hour, minute, second); // output korean standard time : Serial.print(“korean standard time : “); hour = (hour + 9) % 24; // korean standard time = GMT + 9 printTime(hour, minute, second); } Serial.println(); // After 10 seconds, again ask the time to NTP server and output it delay(10000); } // Send an NTP request to the given NTP server address unsigned long sendNTPpacket(char* address) { // Make all the data in the buffer 0 memset(packetBuffer, 0, NTP_PACKET_SIZE); // Initialize the necessary values to create an NTP request message // (Refer to the URL above for more information about the packet) packetBuffer[0] = 0b11100011; // LI, Version, Mode packetBuffer[1] = 0; // Stratum, or type of clock packetBuffer[2] = 6; // Polling Interval packetBuffer[3] = 0xEC; // Peer Clock Precision // 8 bytes of zero for Root Delay & Root Dispersion packetBuffer[12] = 49; packetBuffer[13] = 0x4E; packetBuffer[14] = 49; packetBuffer[15] = 52; // All NTP fields are filled with the given value, // Now we can send packets to get the time stamp: Udp.beginPacket(address, 123); //NTP request to port 123 Udp.write(packetBuffer, NTP_PACKET_SIZE); Udp.endPacket(); } void printTime(int hour, int minute, int second) { print2digits(hour); // Output hour Serial.print(‘:’); print2digits(minute); // Output minute Serial.print(‘:’); print2digits(second); // Output second Serial.println(); } // Output the number as two-digit number void print2digits(int number) { if(number < 10) { Serial.print(‘0’); } Serial.print(number); } |
Colored by WayFarer™ |
3. Execution result
If you upload a sketch and open the serial monitor and wait for a while, the time stamp is received from the NTP server and the seconds and the time are displayed on the screen. If you wait 10 seconds and repeat it again, time after 11 seconds will appear on the screen.
4. Finishing
I do not need any circuit configuration to run this sketch, which is one of the examples I easily tested. Times, minutes, and seconds are simple to print, but it seems to require the Time library for Unix in order to print the date. You have to calculate the leap year and change the date as well as the time to change it to Korea Standard Time.
Expect to see an example of outputting to a local date sometime …
Date : Oct 10, 2014
Author : 과객
COMMENTS