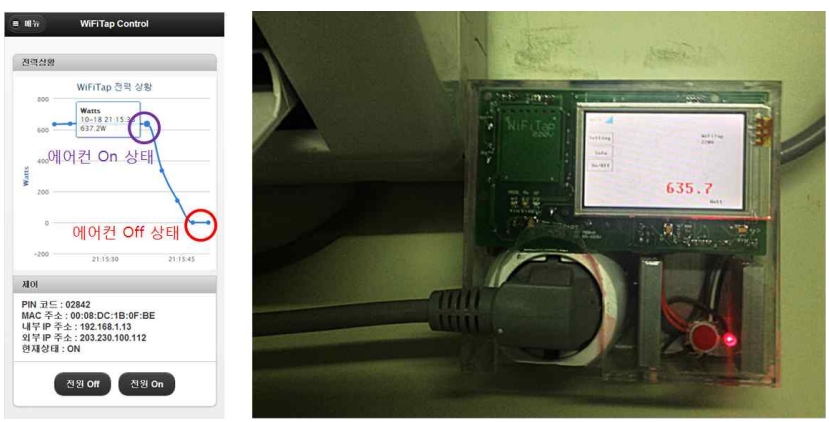
This example is a sketch that prints the year / month / date on a serial monitor using the Time Library i and “Ethernet Shield W5100 Attachment | Udp Ntp Client Example”.
1. Materials and circuits
You will need an Ethernet shield board, an Arduino board or a mega board. You must have an Internet router (router or DHCP server) to be assigned an IP address.
materials
=====
- Arduno board
- Ethernet shield board
- Internet router
- Internet line
Circuit configuration
=======
The circuit configuration is not required, but the Ethernet shield is attached to the Arduino board and connected to the Internet.
2. Sketch Description
Time library uses Unix time, so 1970/1/1 is the base date (start date), and NTP use 1900/1/1 as the base date using UTC (Greenwich Meridian Time). You have to subtract the value from the 1900/1/1 to the 1969/12/31 hours (365 * 70 + 17 (number of leap years)) * 24 hours * 60 minutes * 60 seconds = 2208988800. With this value, I got the year, month, and day using the year (), month (), and day () functions of the Time library.
Also, Korea Standard Time is 9 o’clock faster than UTC, so we changed it to 9 * 60 * 60 = 9 * 3600 seconds in addition to Unix Time.
sketch
=====
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 | /* Udp NTP Client 2 For more information about the NTP time server and the messages it sends and receives Please refer to: http://en.wikipedia.org/wiki/Network_Time_Protocol It was created by Michael Margolis on September 04, 2010, Modified by Tom Igoe on April 09, 2012 This code is a shared asset. */ #include <SPI.h> #include <Ethernet.h> #include <EthernetUdp.h> #include <Time.h> // put in the MAC address for the Ethernet controller // The new Ethernet shield has the MAC address printed on the sticker byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED }; unsigned int localPort = 8888; // local port to receive UDP packets char timeServer[] = “time.nist.gov”; // time.nist.gov NTP server const int NTP_PACKET_SIZE = 48;// Size of the NTP message (packet data) byte packetBuffer[ NTP_PACKET_SIZE];// Buffer for sending and receiving packets // UDP instance (object) to send / receive packets using UDP protocol EthernetUDP Udp; void setup() { // Open the serial communication and wait for the serial communication to be connected: Serial.begin(9600); while (!Serial) { ;// Wait for the serial port to connect. Requires only Leonardo board } // Start Ethernet and UDP if (Ethernet.begin(mac) == 0) { Serial.println(“Failed to configure Ethernet using DHCP”); // no more to run and infinite looping:` for (;;) ; } Udp.begin(localPort); } void loop() { sendNTPpacket(timeServer); // Send an NTP packet to the time server // Check if the response came delay(1000); if ( Udp.parsePacket() ) { // Get the data from the received response packet Udp.read(packetBuffer, NTP_PACKET_SIZE);// Load the packet into the buffer // The timestamp starts at the 40th byte of the received packet and consists of 4 bytes (2 // word or long). 2 Separate word data. unsigned long highWord = word(packetBuffer[40], packetBuffer[41]); unsigned long lowWord = word(packetBuffer[42], packetBuffer[43]); // Create a integer in long type by combining 4byte(2word) // NTP time (seconds since January 1, 1900 to the present) // calculate): unsigned long secsSince1900 = highWord << 16 | lowWord; Serial.print(“seconds(秒) time : ” ); Serial.println(secsSince1900); // Convert NTP time to day time: Serial.print(“Unix time : “); //Unix time starts on January 1, 1970, and is calculated in seconds //2208988800 is: const unsigned long seventyYears = 2208988800UL; // Subtract the corresponding seconds in 70 years: unsigned long epoch = secsSince1900 – seventyYears; // Output the Unix time: Serial.println(epoch); int hour, minute, second; // Print Coordinated Universal Time:: Serial.print(“Coordinated Universal Time: : “); // UTC is Greenwich // Meridian Time:GM. hour = (epoch % 86400L) / 3600;// 1day = 86400sec, 1h = 3600sec minute = (epoch % 3600) / 60; // 1h = 3600sec, 1m = 60sec second = epoch % 60; // 1m = 60sec printTime(hour, minute, second); Serial.print(” “); printDate(year(epoch), month(epoch), day(epoch)); Serial.println(); // output Korean Standard Time: Serial.print(” Korean Standard Time : “); hour = (hour + 9) % 24; // Korean Standard Time = GMT + 9 printTime(hour, minute, second); epoch += 9 * 3600; // GMT + 9 * 60 * 60 Serial.print(” “); printDate(year(epoch), month(epoch), day(epoch)); Serial.println(); } Serial.println(); // After 10 seconds, ask the time again from the NTP server and output it delay(10000); } // Send an NTP request to the given NTP server address unsigned long sendNTPpacket(char* address) { // Make all the data 0 in the buffer memset(packetBuffer, 0, NTP_PACKET_SIZE); // Initialize the necessary values to create an NTP request message // (See the URL above for more information about the packet) packetBuffer[0] = 0b11100011; // LI, Version, Mode packetBuffer[1] = 0; // Stratum, or type of clock packetBuffer[2] = 6; // Polling Interval packetBuffer[3] = 0xEC; // Peer Clock Precision // 8 bytes of zero for Root Delay & Root Dispersion packetBuffer[12] = 49; packetBuffer[13] = 0x4E; packetBuffer[14] = 49; packetBuffer[15] = 52; // All NTP fields are filled with the given value, // Now we can send the packet to get timestampt: Udp.beginPacket(address, 123); // NTP request to port 123 Udp.write(packetBuffer, NTP_PACKET_SIZE); Udp.endPacket(); } // print hour, minute and second void printTime(int hour, int minute, int second) { print2digits(hour); Serial.print(‘:’); print2digits(minute); Serial.print(‘:’); print2digits(second); } // Print year, month, and date void printDate(int year, int month, int day) { Serial.print(year); Serial.print(“/”); Serial.print(month); Serial.print(“/”); Serial.print(day); } // Output the number as two-digit number void print2digits(int number) { if(number < 10) { Serial.print(‘0’); } Serial.print(number); } |
Colored by WayFarer™ |
3. Execution result
When I pressed the button to upload the sketch, an error occurred in the time library that I used well. It seems that the problem of using flash memory as a data area after upgrading to Arduino IDE 1.5.8 is a little more strict. Based on the error message, modify the Time library to upload successfully, open the serial monitor window, and finally the desired date will start to appear on the screen.
For reference, place the URL of the Time library and the modified DateStrings.cpp library file in the attachment.
Time Library: http://playground.arduino.cc/Code/Time
Date : Oct 21, 2014
Author : 과객
COMMENTS