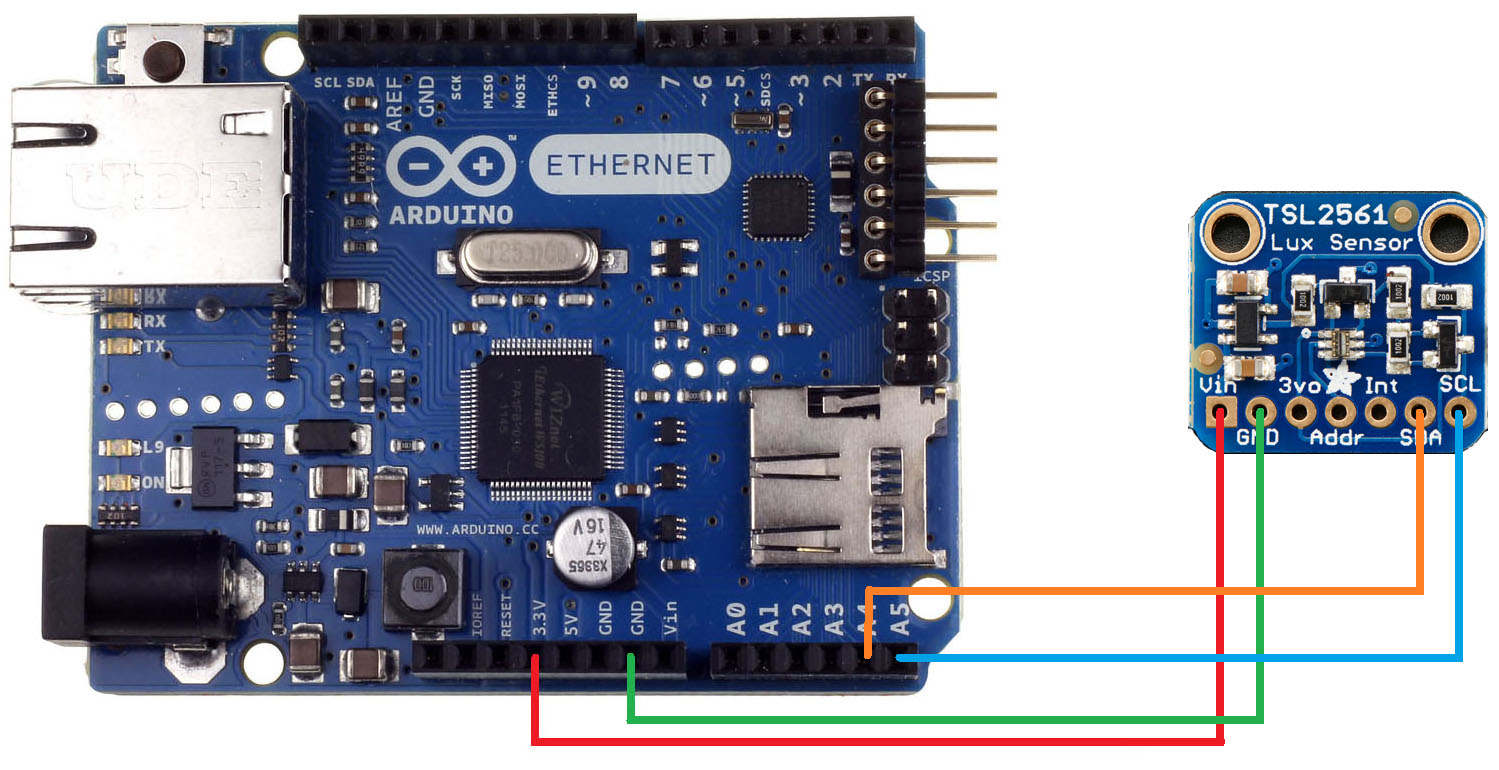
details
This project allows you to check the luminance from a TSL2561 luminance sensor on a mobile App.
Hardware:
Arduino Uno
Ethernet Shield
Luminanace Sensor(TSL2561)
Wires.
Wiring
This section shows you how to connect the components together. First you have a pin-out connection and below there are pictures showing how it’s done on the real boards.
Luminance Sensor | Pin ArduinoUno |
GND | GND |
Vin | +5V |
SDA | A4 |
SCL | A5 |
The schematic is
#include <Wire.h> #include <Adafruit_Sensor.h> #include <Adafruit_TSL2561_U.h> #include <SPI.h> #include <Ethernet.h> byte mac[] = { 0xDE, 0xED, 0xBE, 0xEF, 0xFE, 0xD2 }; // mac address for ethernet board EthernetClient eth; // the Ethernet client char server[] = "www.ic2pro.com"; // Wiring Cloud host name int port = 80; String devId = "111-222-333"; // Device ID. CREATE YOUR OWN GUID; Use this http://www.guidgenerator.com/ String auth = "dXNlcjpwYXNzd29yZA=="; // Authentication credentials Create a string from <email_address>:<API_Password> and encode it base64 // The sample here "dXNlcjpwYXNzd29yZA==" is the encoding for "user:password" Adafruit_TSL2561_Unified tsl = Adafruit_TSL2561_Unified(TSL2561_ADDR_FLOAT, 12345); // Update LUMINANCE signal on the Wiring Cloud void sendLuminance(String luminance) { Serial.println("Luminance = " + luminance); Serial.println("Send Luminance..."); if (eth.connect(server, port)) { eth.println("GET http://" + String(server) + ":" + String(port) + "/Wire/connector/set?id=" + devId + "&LUMINANCE=" + luminance+ " HTTP/1.1"); eth.println("Authorization: Basic " + auth); eth.println("Connection: close"); eth.println(); Serial.println("Luminance sent to server."); } else { Serial.println("Connection Error"); } eth.stop(); } // retrieve luminance from sensor (Check Adafruit documentation to see how it's done) String getLuminance() { String rs = ""; sensors_event_t event; tsl.getEvent(&event); if (event.light) rs = String(event.light); else rs = "N/A"; Serial.print("Luminance: "); Serial.println(rs); return rs; } void displaySensorDetails(void) { sensor_t sensor; tsl.getSensor(&sensor); Serial.println("------------------------------------"); Serial.print ("Sensor: "); Serial.println(sensor.name); Serial.print ("Driver Ver: "); Serial.println(sensor.version); Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id); Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println(" lux"); Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println(" lux"); Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println(" lux"); Serial.println("------------------------------------"); Serial.println(""); delay(500); } void configureSensor(void) { tsl.enableAutoRange(true); // Auto-gain ... switches automatically between 1x and 16x tsl.setIntegrationTime(TSL2561_INTEGRATIONTIME_13MS); // fast but low resolution /* Update these values depending on what you've set above! */ Serial.println("------------------------------------"); Serial.print ("Gain: "); Serial.println("Auto"); Serial.print ("Timing: "); Serial.println("13 ms"); Serial.println("------------------------------------"); } void setup() { Serial.begin(9600); Serial.println("Start..."); Ethernet.begin(mac); // start the Ethernet connection: delay(1000); // give the Ethernet shield a second to initialize: if(!tsl.begin()) // check if luminance sensor is workign { Serial.print("Ooops, no TSL2561 detected ... Check your wiring or I2C ADDR!"); while(1); } /* Display some basic information on this sensor */ displaySensorDetails(); /* Setup the sensor gain and integration time */ configureSensor(); } void loop() { sendLuminance(getLuminance()); delay(5000); // send luminance only every 5 seconds }
Final Product from the app:
Tags:201802,Arduino Uno,Ethernet Shield w5100,Luminance Sensor.
Original Source: https://www.ic2cloud.com/luminance-iot
COMMENTS