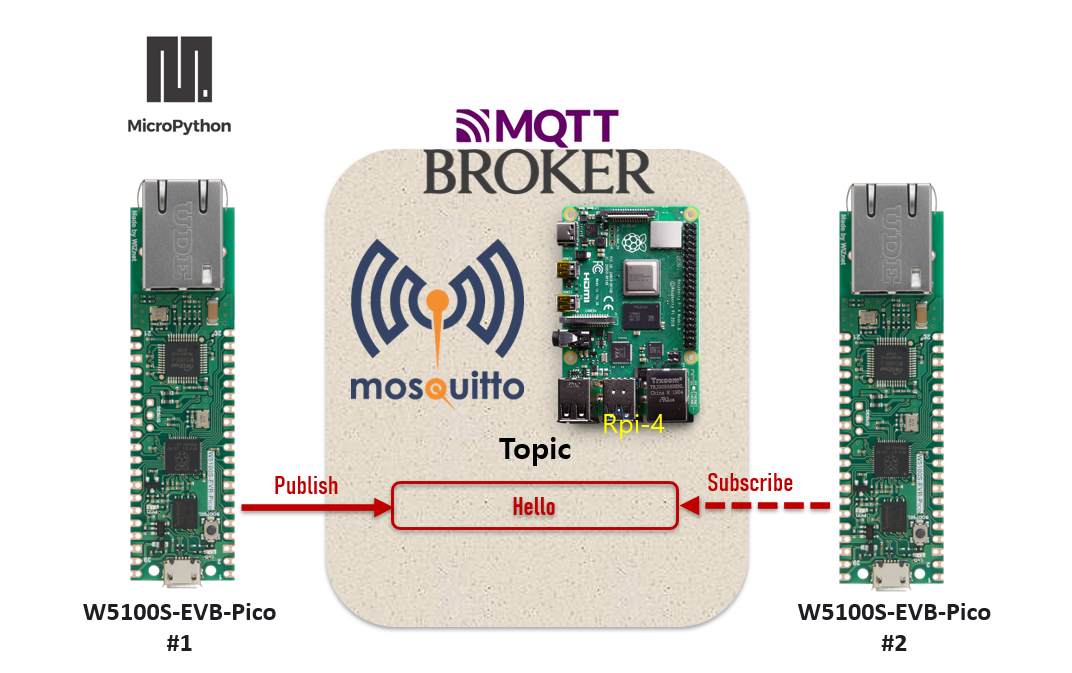
MQTT?
The pub/sub model and subscriber/subscriber model are communication models between subscription clients and issuance clients.
- Lightweight message protocols created for use in M2M and the Internet of Things.
- Operation on TCP
- <Publisher> issues Topic.
- <Subscriber> subscribes to Topic.
- <Broker> serves as a relay and is suitable for 1:N communication.
- Suitable for messaging processing of “device to devices” such as low power power sensors, mobile devices, embedded computers, Arduinoes, and microcontrollers.
Overview
WIZnet Ethernet HAT (Hardware Attached on Top) is a Raspberry Pi Pico pin-compatible board that utilizes W5100S and supports both 3.3V & 5V.
Please refer to this link to find more information about W5100S.
- Raspberry Pi Pico Pin-Compatible
- Ethernet (W5100S Hardwired TCP/IP CHIP)
- Product page : https://docs.wiznet.io/Product/iEthernet/W5100S/overview
- Support 4 Independent Hardware SOCKETs simultaneously
- Support SOCKET-less new Command: ARP-Request, PING-Request
- Support Auto-MDIX only when Auto-Negotiation mode
RP2040 Datasheet – https://www.raspberrypi.org/documentation/microcontrollers/raspberry-pi-pico.html
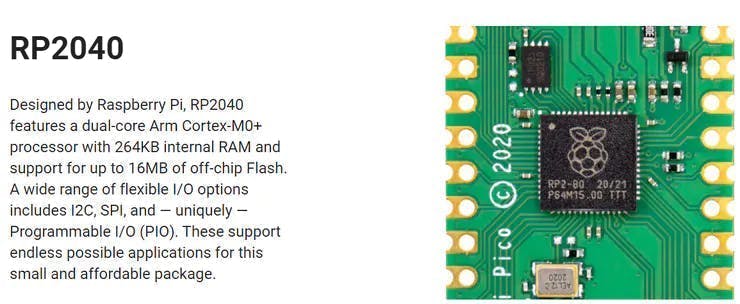
Prepare
Connect Raspberry Pi
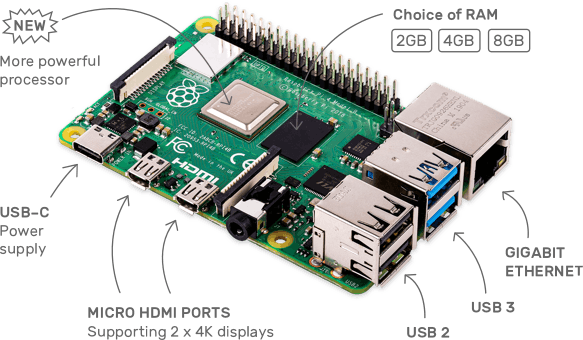
Power the power supply and connect the Ethernet cable.
Run RPi-4 Mosquitto Server.
After having your Raspberry Pi board prepared with Raspberry Pi OS, you can continue with this tutorial. Let’s install the Mosquitto Broker.
1. Raspberry Pi opens the terminal window through SSH.
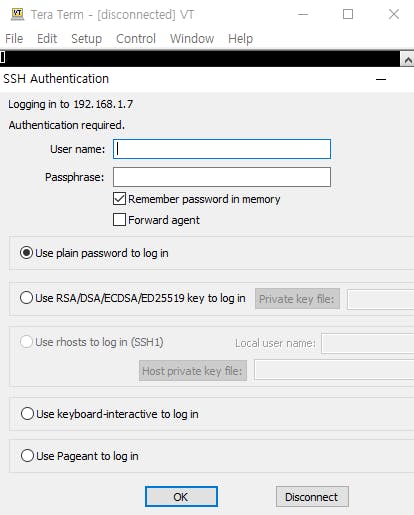
2.Run the following command to upgrade and update your system:
pi@raspberry:~ $ sudo apt update && sudo apt upgrade
3. Press Y and Enter. It will take some time to update and upgrade (in my case, it took approximately 10 minutes).
4. To install the Mosquitto Broker enter these next commands:
pi@raspberry:~ $ sudo apt install -y mosquitto mosquitto-clients
5. To make Mosquitto auto start when the Raspberry Pi boots, you need to run the following command (this means that the Mosquitto broker will automatically start when the Raspberry Pi starts):
pi@raspberry:~ $ sudo systemctl enable mosquitto.service
6. Now, test the installation by running the following command:
pi@raspberry:~ $ mosquitto -v
7. Check if the Mosquitto server is operating with the command below. You can also stop and start the server with commands.
Status
pi@raspberry:~ $service mosquitto status
Stop
pi@raspberry:~ $service mosquitto stop
Start
pi@raspberry:~ $service mosquitto start
If you check the status as below, the server will operate normally.
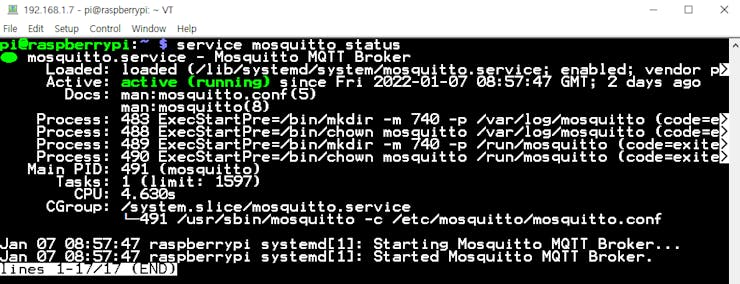
Connect W5100S-Pico-EVB
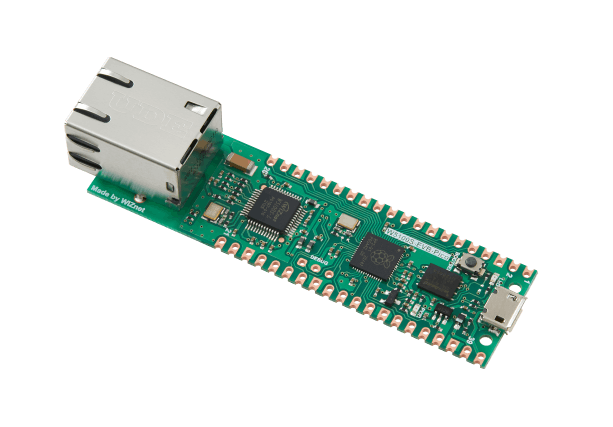
Connect Raspberry Pi Pico to desktop or laptop using 5 pin micro USB cable.
Development environment configuration
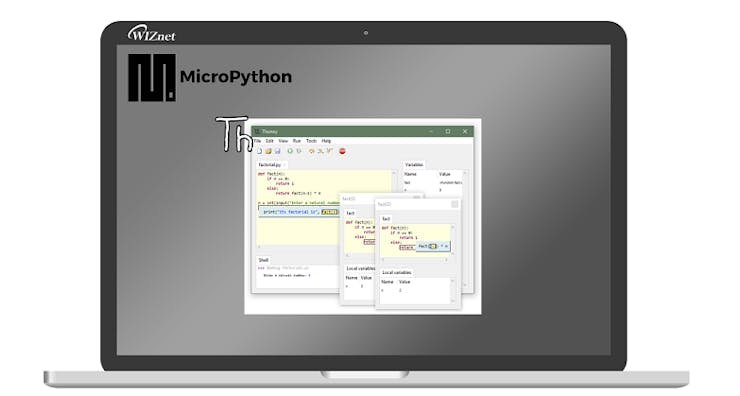
Install Thonny IDE
on Raspberry Pi Pico by referring to the link above.👇
1. The drive will be called RPI-RP2 on all RP2040 boards. Download the UF2(firmware.uf2) file from the link below and put the file in Pico.
File link – https://github.com/Wiznet/RP2040-HAT-MicroPython/releases/tag/v1.0.2
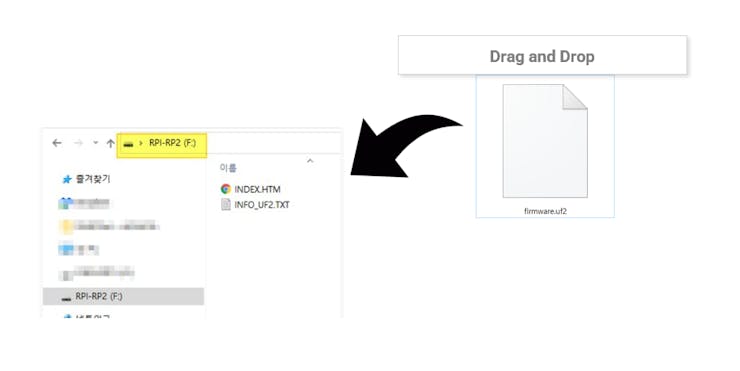
2. You can also access the firmware installation menu if you click on MicroPython (Raspberry Pi Pico) in the status bar and choose ‘Configure interpreter …’.
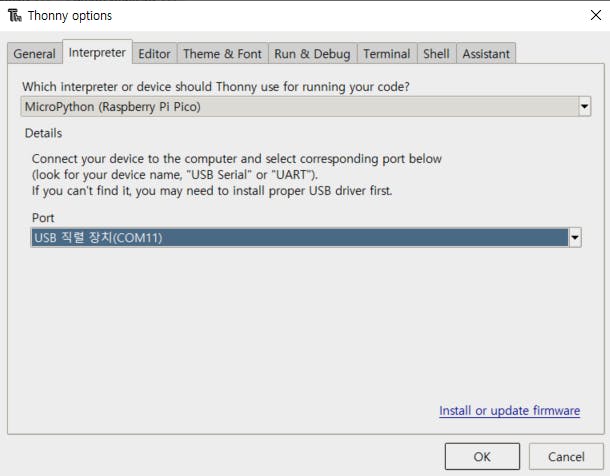
3. Look at the Shell panel at the bottom of the Thonny editor. You should see something like this:
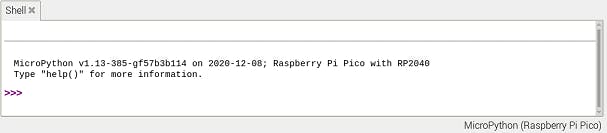
Importing MQTT library
First, import the library of the function you want to use from the library to your PC. To uploading a file to using Thonny IDE, follow these next steps.
Micropython Ethernet Libraries
- Create a new file, Save it in your computer with the exact name that you want, for example “(your library name).py”
- Go to Open > This computer
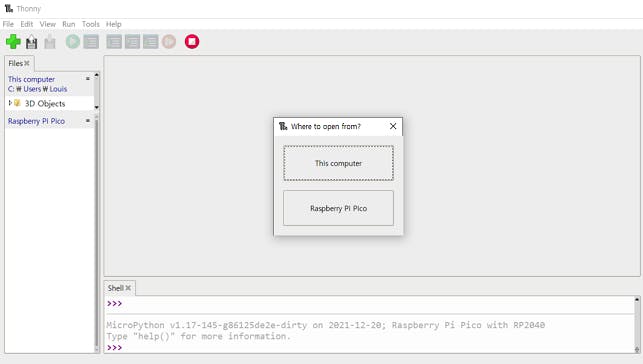
The file should be saved on RPi Pico with the name “(your library name).py”
- Go to File > Save as > Raspberry Pi Pico
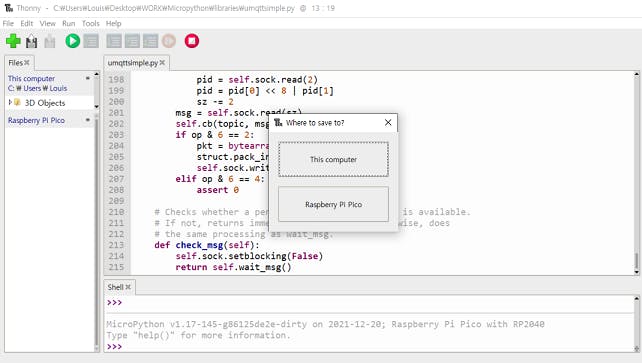
Publish
#### MQTT Pico (1) ##### – publish
1. Setup SPI interface and Initialize ethernet configuration.
spi=SPI(0,2_000_000, mosi=Pin(19),miso=Pin(16),sck=Pin(18))
nic = network.WIZNET5K(spi,Pin(17),Pin(20)) #spi,cs,reset pin
nic.ifconfig(('192.168.1.20','255.255.255.0','192.168.1.1','8.8.8.8'))
while not nic.isconnected():
time.sleep(1)
print(nic.regs())
2.In the MQTT configuration, the broker IP address is the IP of your Raspberry Pi server.
#mqtt config
mqtt_server = '192.168.1.7'
client_id = 'wiz1'
topic_pub = b'hello'
topic_msg = b'Hello Pico'
last_message = 0
message_interval = 5
counter = 0
#MQTT connect
def mqtt_connect():
client = MQTTClient(client_id, mqtt_server, keepalive=60)
client.connect()
print('Connected to %s MQTT Broker'%(mqtt_server))
return client
#reconnect & reset
def reconnect():
print('Failed to connected to MQTT Broker. Reconnecting...')
time.sleep(5)
machine.reset()
3. going to use MQTT Publish.
#MQTT Publish
def main():
w5x00_init()
try:
client = mqtt_connect()
except OSError as e:
reconnect()
while True:
client.publish(topic_pub, topic_msg)
time.sleep(3)
client.disconnect()
Sending a “Hello WIZnet” message to the mosquitto server with the topic “Hello”.
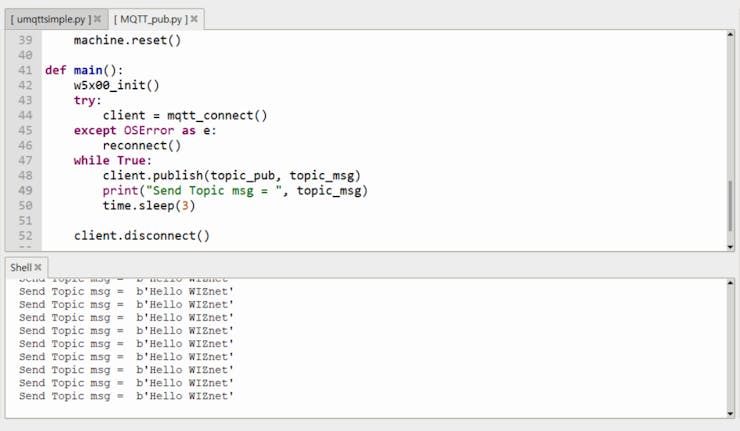
Subscribe
#### MQTT Pico (2) ##### – subscribe
1. Setup SPI interface and Initialize ethernet configuration.
spi=SPI(0,2_000_000, mosi=Pin(19),miso=Pin(16),sck=Pin(18))
nic = network.WIZNET5K(spi,Pin(17),Pin(20)) #spi,cs,reset pin
nic.ifconfig(('192.168.1.20','255.255.255.0','192.168.1.1','8.8.8.8'))
while not nic.isconnected():
time.sleep(1)
print(nic.regs())
2. In the MQTT configuration, the broker IP address is the IP of your Raspberry Pi server.
#mqtt config
mqtt_server = '192.168.1.7'
client_id = "wiz"
topic_sub = b'hello'
last_message = 0
message_interval = 5
counter = 0
def sub_cb(topic, msg):
print((topic.decode('utf-8'), msg.decode('utf-8')))
#MQTT connect
def mqtt_connect():
client = MQTTClient(client_id, mqtt_server, keepalive=60)
client.set_callback(sub_cb)
client.connect()
print('Connected to %s MQTT Broker'%(mqtt_server))
return client
#reconnect & reset
def reconnect():
print('Failed to connected to Broker. Reconnecting...')
time.sleep(5)
machine.reset()
3. going to use MQTT Subscribe.
#subscribe
def main():
w5x00_init()
try:
client = mqtt_connect()
except OSError as e:
reconnect()
while True:
client.subscribe(topic_sub)
time.sleep(1)
client.disconnect()
If you subscribe to “Hello” topic, you can get a message from Publisher.
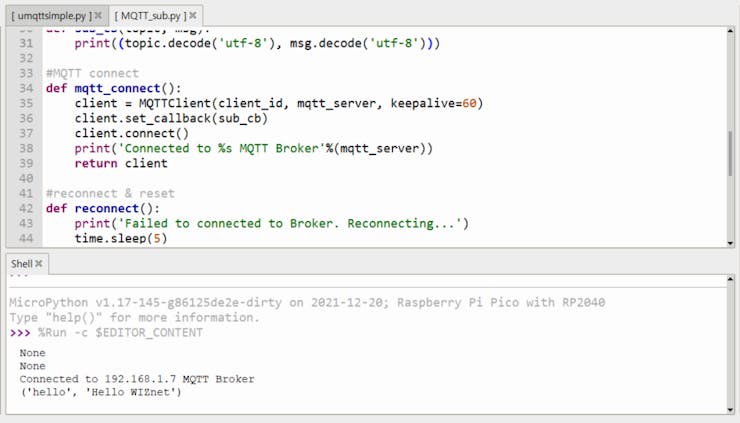
COMMENTS